Warning
After SDK version 1.4.4, it is required to use Gradle 8 or later, so you must update the JDK version to 17 to be compatible
JDK Upgrade
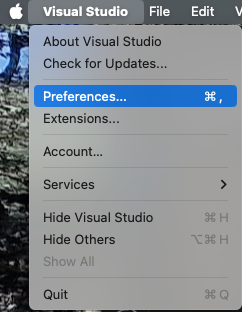
If you do not have the version through Android Studio, you can download it from here: JDK 17 oracle
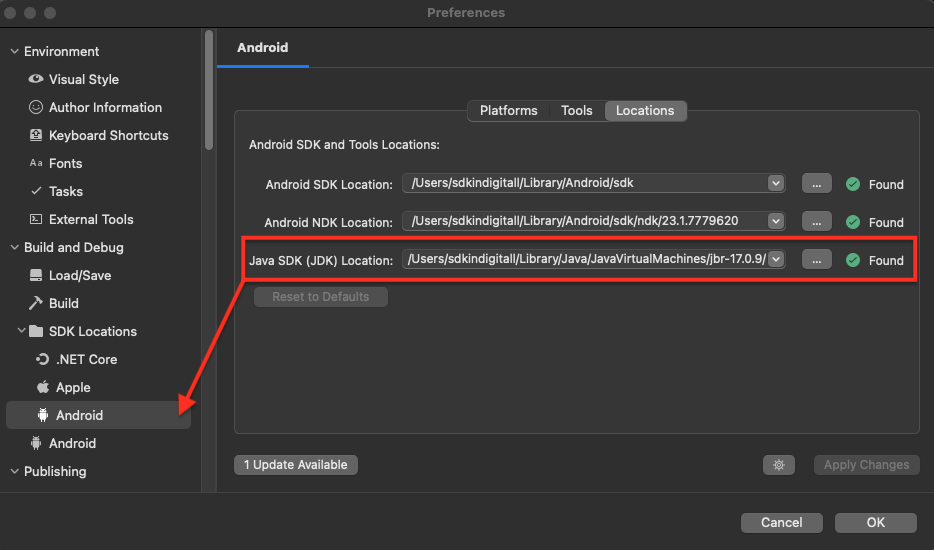
Adding Firebase Services
-
To start you need a file called google-services.json. This file can be exported from the Firebase console. Move it to the root folder of your project.
-
Add the services from indigitall to your AndroidManifest.xml as you can see below.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android"> <application android:allowBackup="true" android:icon="@mipmap/launcher_foreground" android:supportsRtl="true"> <service android:name="Com.Huawei.Hms.Location.LocationServices" android:exported="false"></service> <service android:name="com.indigitall.android.push.services.StatisticService" android:exported="false" /> <service android:name="com.indigitall.android.push.services.NightService" /> <receiver android:name="com.indigitall.android.push.receivers.BootReceiver" android:exported="false"> <intent-filter><action android:name="android.intent.action.BOOT_COMPLETED" /></intent-filter> </receiver> <receiver android:name="com.indigitall.android.push.receivers.LocationReceiver" android:exported="false"> <intent-filter><action android:name="LocationReceiver.Action.LOCATION_UPDATE" /></intent-filter> </receiver> <receiver android:exported="false" android:name="com.indigitall.android.push.receivers.AlarmReceiver"> <intent-filter><action android:name="AlarmReceiver.Action.NETWORK_ALARM" /></intent-filter> </receiver> <service android:name="com.indigitall.android.push.services.FirebaseMessagingService" android:exported="false"> <intent-filter><action android:name="com.google.firebase.MESSAGING_EVENT" /></intent-filter> </service> <service android:name="com.indigitall.android.push.services.WifiStatusService" android:permission="android.permission.BIND_JOB_SERVICE" android:exported="false"></service> </application> <uses-permission android:name="android.permission.POST_NOTIFICATIONS" /> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" /> <uses-permission android:name="android.permission.VIBRATE" /> <uses-permission android:name="android.permission.WAKE_LOCK" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_BACKGROUND_LOCATION" /> <uses-permission android:name="android.permission.CHANGE_WIFI_STATE" /> <uses-permission android:name="android.permission.ACCESS_WIFI_STATE" /> <!-- ANDROID 12 WIFI--> <uses-permission android:name="android.permission.CHANGE_NETWORK_STATE" /> <uses-sdk android:minSdkVersion="21" android:targetSdkVersion="33" /> </manifest>
Push permission after Android 13 (Api level 33 - Tiramisu)Override onRequestPermissionsResult in the Main Activity:
public override void OnRequestPermissionsResult(int requestCode, string[] permissions, Permission[] grantResults) { new Com.Indigitall.Maui.Platforms.Android.MIndigitall().OnRequestPermissionsResult(requestCode, permissions, grantResults); }
Adding HMS ServicesHuawei currently does not support the Maui SDK.
Before you start, you have to add the following nuget package: Com.Indigitall.Xamarin.Hms
-
To start you need a file called agconnect-services.json. This file can be exported from the Huawei developer console.
-
Copy agconnect-services.json into the Assets folder of your project.
-
Create a file HmsLazyInputStream.cs in the root of the project that will be used to read the content of agconnect-services.json.
-
Add the following code in the AttachBaseContext of the MainActivity to call HmsLazyInputStream.cs:
protected override void AttachBaseContext(Context context) { base.AttachBaseContext(context); AGConnectServicesConfig config = AGConnectServicesConfig.FromContext(context); config.OverlayWith(new HmsLazyInputStream(context)); }
- Add the following code in HmsLazyInputStream.cs
using System; using System.IO; using Android.Content; using Android.Util; using Com.Huawei.Agconnect.Config; namespace XamarinDemo.Droid { public class HmsLazyInputStream : LazyInputStream { public HmsLazyInputStream(Context context) : base(context) { } public override Stream Get(Context context) { try { return context.Assets.Open("agconnect-services.json"); } catch (Exception e) { Log.Error(e.ToString(), "Can't open agconnect file"); return null; } } } }
- Add the HMS services indigitall in your AndroidManifest.xml.
<!--HMS Services START--> <service android:name="Com.Huawei.Hms.Location.LocationServices"></service> <service android:name="com.indigitall.android.hms.services.HMSMessagingService" android:exported="false"> <intent-filter><action android:name="com.huawei.push.action.MESSAGING_EVENT" /></intent-filter> </service> <!--HMS Services END-->
-