This integration has been done with the IDE Visual Studio.
You can see it in this tutorial video or read the instructions below:
JDK Upgrade
Warning
After SDK version 3.0.0, it is required to use Gradle 8 or later, so you must update the JDK version to 17 to be compatible
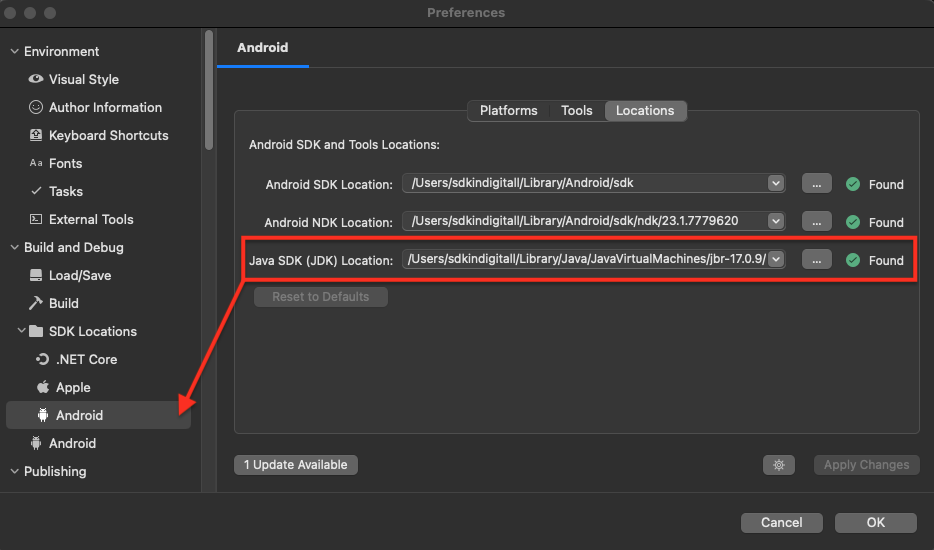
If you do not have the version through Android Studio, you can download it from here: JDK 17 oracle
Adding Firebase Services
-
- To start you need a file called google-services.json. This file can be exported from the Firebase console. Move it to the root folder of your project.
-
- Add the services from indigitall to your AndroidManifest.xml as you can see below.
<manifest ...>
<!-- ... -->
<!-- START indigitall permissions -->
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" />
<uses-permission android:name="android.permission.VIBRATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<!-- END indigitall permissions -->
<application ...>
<!-- ... -->
<!-- START indigitall services -->
<service android:name="com.indigitall.android.push.services.StatisticService" />
<service android:name="com.indigitall.android.push.services.NightService" />
<receiver android:name="com.indigitall.android.push.receivers.BootReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED" />
</intent-filter>
</receiver>
<receiver android:name="com.indigitall.android.push.receivers.LocationReceiver">
<intent-filter>
<action android:name="LocationReceiver.Action.LOCATION_UPDATE" />
</intent-filter>
</receiver>
<service android:name="com.indigitall.android.push.services.FirebaseMessagingService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
<meta-data android:name="indigitall.color" android:resource="@color/colorprimary" />
<meta-data android:name="indigitall.icon" android:resource="@mipmap/launcher_foreground" />
<!-- END indigitall services -->
</application>
</manifest>
Push permission after Android 13 (Api level 33 - Tiramisu)
Android 13 requires you to add the android.permission.POST_NOTIFICATIONS flag to the manifest:
<uses-permission android:name="android.permission.POST_NOTIFICATIONS"/>
And also override onRequestPermissionsResult in the Main Activity:
public override void OnRequestPermissionsResult(int requestCode, string[] permissions, [GeneratedEnum] Permission[] grantResults)
{
base.OnRequestPermissionsResult(requestCode, permissions, grantResults);
Com.Indigitall.Xamarin.Android.Utils.PermissionUtils.OnRequestPermissionResult(this, requestCode, permissions, grantResults);
}
Adding HMS Services
Before you start, you have to add the following nuget package: Com.Indigitall.Xamarin.Hms
-
To start you need a file called agconnect-services.json. This file can be exported from the Huawei developer console.
-
Copy agconnect-services.json into the Assets folder of your project.
-
Create a file HmsLazyInputStream.cs in the root of the project that will be used to read the content of agconnect-services.json.
- Add the following code in the AttachBaseContext of the MainActivity to call HmsLazyInputStream.cs:
protected override void AttachBaseContext(Context context)
{
base.AttachBaseContext(context);
AGConnectServicesConfig config = AGConnectServicesConfig.FromContext(context);
config.OverlayWith(new HmsLazyInputStream(context));
}
- Add the following code in HmsLazyInputStream.cs
using System;
using System.IO;
using Android.Content;
using Android.Util;
using Com.Huawei.Agconnect.Config;
namespace XamarinDemo.Droid
{
public class HmsLazyInputStream : LazyInputStream
{
public HmsLazyInputStream(Context context) : base(context)
{
}
public override Stream Get(Context context)
{
try
{
return context.Assets.Open("agconnect-services.json");
}
catch (Exception e)
{
Log.Error(e.ToString(), "Can't open agconnect file");
return null;
}
}
}
}
- Add the following HMS NuGet packages to have the push and location services in your application:
- Add the HMS services indigitall in your AndroidManifest.xml.
<!--HMS Services START-->
<service android:name="Com.Huawei.Hms.Location.LocationServices"></service>
<service android:name="com.indigitall.android.hms.services.HMSMessagingService" android:exported="false">
<intent-filter>
<action android:name="com.huawei.push.action.MESSAGING_EVENT" />
</intent-filter>
</service>
<!--HMS Services END-->